Okay, so today I decided to mess around with something I called “famous initials”. It wasn’t anything groundbreaking, just a little exercise I set for myself to keep the gears turning, you know?
Getting Started
First thing, I grabbed my laptop and opened up my usual text editor. The idea was pretty simple: take a list of famous people’s names and pull out just their initials. Seemed straightforward enough for a quick practice session.
I started by jotting down a few names that popped into my head. Just typed them out like this:
- Albert Einstein
- Isaac Newton
- Marie Curie
- Leonardo da Vinci
- Stephen Hawking
Nothing fancy, just a basic list to work with. I figured I’d put these into a list in my little script later.
Figuring Out the Steps
Alright, names in hand, I thought, “How do I actually get the initials?” My first thought was, okay, each name has a first part and a last part, usually. Sometimes more, like ‘da Vinci’.
So, the plan became:
- Take a full name string (like “Albert Einstein”).
- Split it up where the space is. That should give me [“Albert”, “Einstein”].
- Grab the very first letter of “Albert” (which is ‘A’).
- Grab the very first letter of “Einstein” (which is ‘E’).
- Stick ’em together. A. E. Maybe with periods, maybe not. Decided to just go for ‘AE’ first, keep it simple.
Seemed like a solid plan. Time to actually write some code.
Doing the Work
I decided to use Python because it’s pretty quick for this kind of text stuff. I put my list of names into a Python list:
names = ['Albert Einstein', 'Isaac Newton', 'Marie Curie', 'Leonardo da Vinci', 'Stephen Hawking']
Then, I needed to go through each name in the list. A simple loop would do the trick. Inside the loop, I’d do the splitting and grabbing.
My first attempt looked something like this (in my head, then typed out): For each name, use the split()
function. That breaks the string into a list of words. So “Albert Einstein” becomes ['Albert', 'Einstein']
.
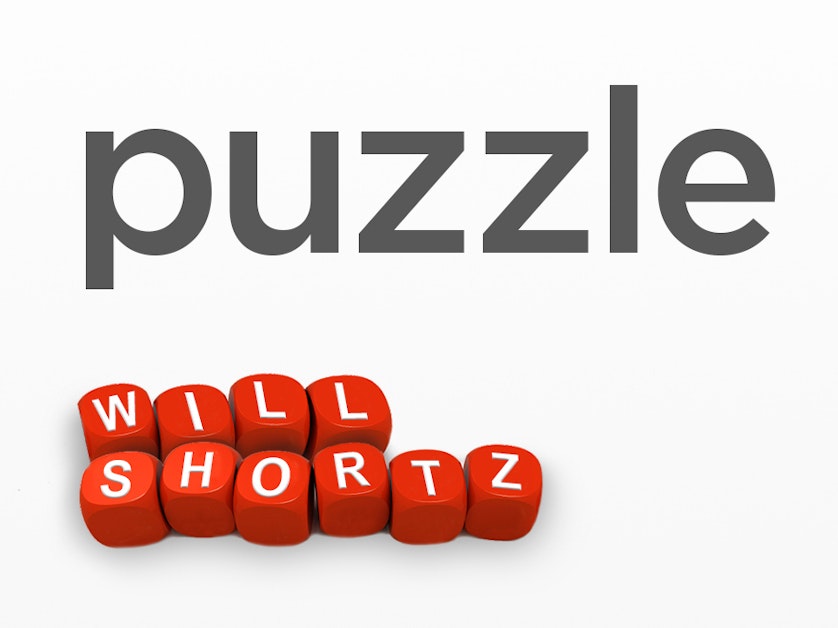
Then, for each word in that new list, I just needed the character at index 0, the first letter. I could build up the initials string piece by piece.
So, inside the loop for each name:
- Split the name:
parts = *(' ')
- Create an empty string for the initials:
initials = ""
- Loop through the
parts
: For eachpart
, addpart[0]
to theinitials
string. - Print the result for that name.
Ran it. And yeah, it mostly worked!
Albert Einstein became AE
Isaac Newton became IN
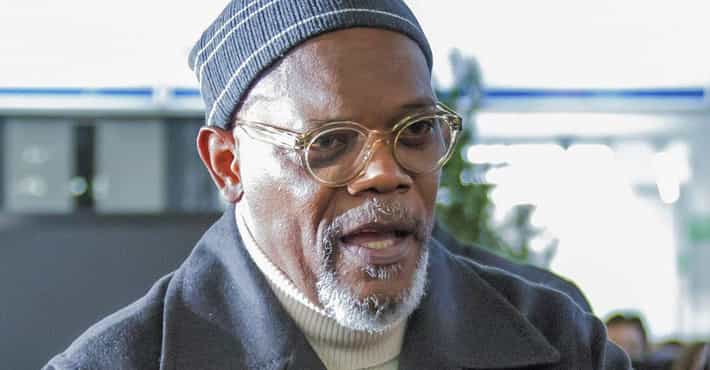
Marie Curie became MC
Leonardo da Vinci became LdV – huh, okay, handles middle/multiple names too. Cool.
Stephen Hawking became SH
Wrapping Up
So yeah, that was the little practice for today. It wasn’t complex, but it was a good way to quickly go through the process: idea, plan, code, test. It felt good to just build something small and see it work right away. Just a simple loop, some string splitting, and accessing characters. Basic stuff, but satisfying.
Didn’t run into many problems, honestly. It went smoother than I thought. Sometimes the simple exercises are the most enjoyable. Gets the job done.
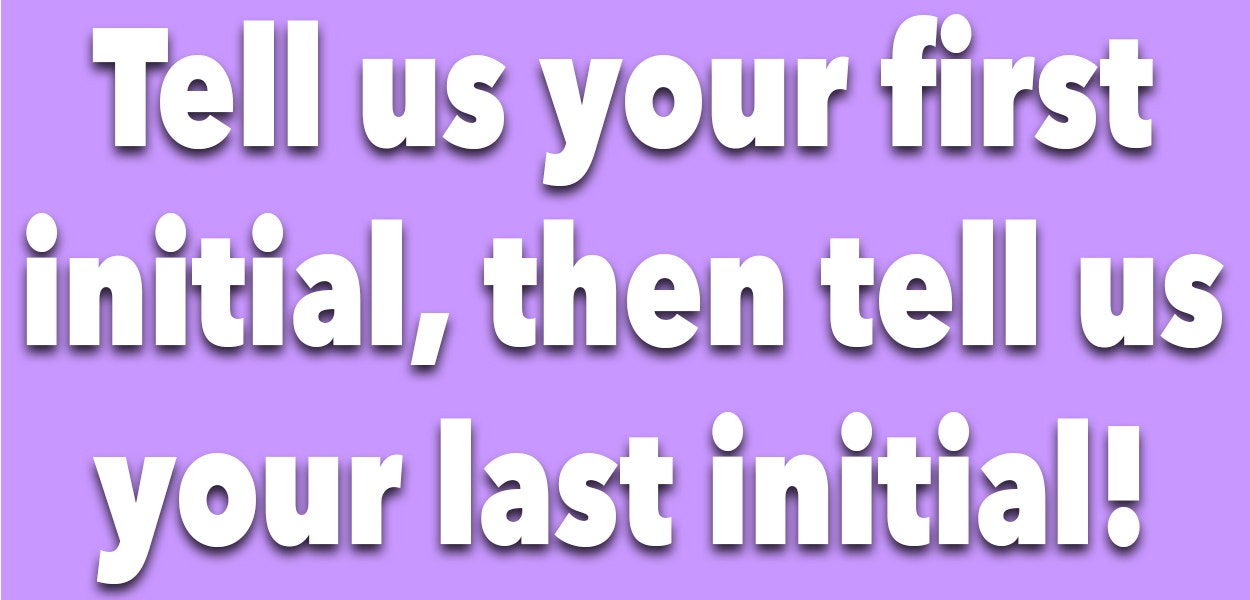