Okay, here’s my take on sharing my “nakedtwins” practice, blog-style:
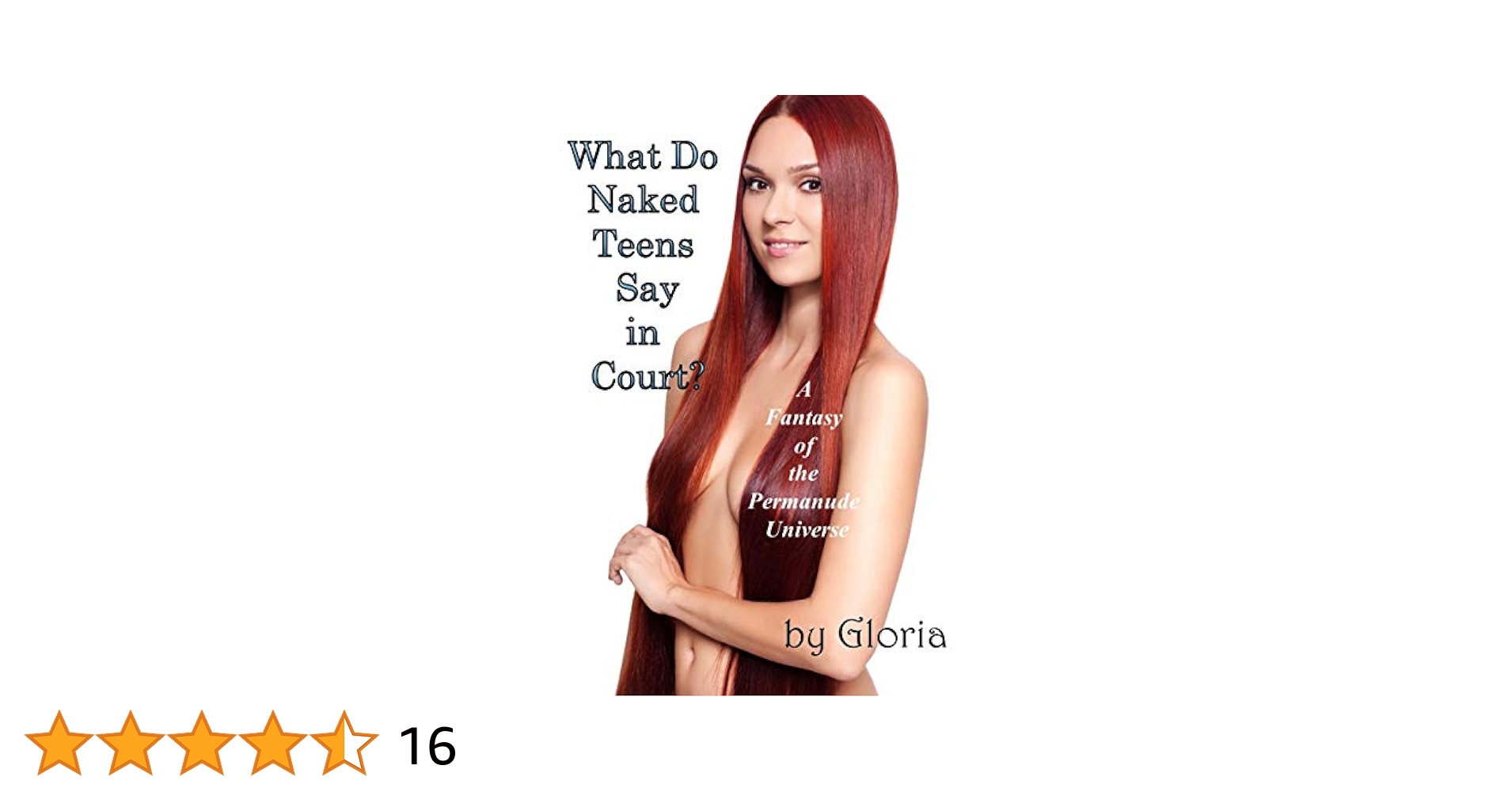
Alright folks, so you know I’ve been messing around with solving Sudoku puzzles programmatically for a while now. And today, I’m gonna walk you through how I implemented the “naked twins” technique. It’s not as scandalous as it sounds, trust me! It’s actually a pretty neat little logic trick.
First off, what are naked twins? Basically, it’s when you have two cells in the same row, column, or 3×3 box that both contain the exact same two possible numbers. And because of that, you know those two numbers can’t be anywhere else in that row, column, or box. They’re stuck in those two cells.
So, where did I even start? Well, I already had a Sudoku solver framework set up. It could handle basic solving, like filling in cells with only one possible value. The next step was to start implementing these more advanced techniques like naked twins.
The very first thing I did was create a function specifically to find possible naked twins.
Here’s a breakdown of how I tackled it:
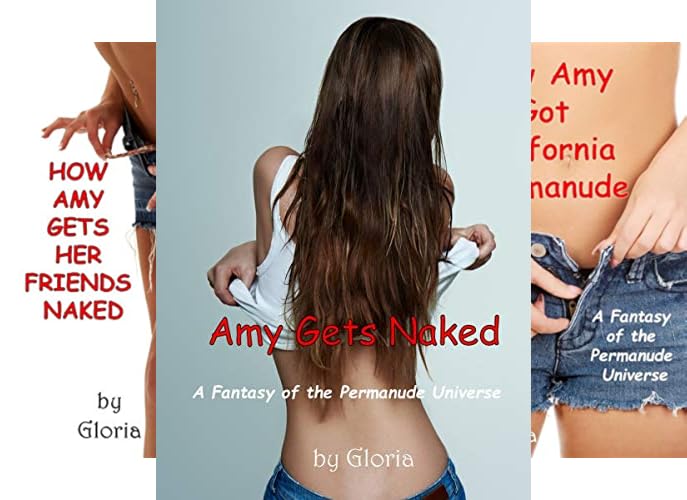
- Iterate: Start by iterating over each row, then column, then each of the 9 blocks.
- Get Possible Values: For each unit(row/col/block), grab the cells that have more than one possible value. This is where the candidates for naked twins live.
- Compare, Compare, Compare: For each cell, compare its possible values with all the other cells in the same unit. If you find two cells with identical possible values (and only two possible values total!), BAM! You’ve got naked twins.
Okay, finding them is one thing. The real fun begins when you start eliminating possibilities. Once I identified a set of naked twins, I had to go back through that same row, column, or box, and remove those two numbers as possibilities from every other cell (except the twins themselves, of course!).
This part was a bit fiddly. Gotta make sure you’re not accidentally removing the numbers from the twin cells, and that you’re correctly updating the possible values for all the other cells.
To make sure everything was running smoothly, I added a bunch of print statements. Nothing fancy, just spitting out the state of the grid before and after applying the naked twins technique, along with the coordinates of the twin cells and the numbers I was removing.
I ran it against some of the tougher puzzles I had lying around, and, you know what? It worked! It wasn’t a magic bullet – some puzzles still needed other techniques. But it definitely helped solve some puzzles that were previously unsolvable with just the basic single-candidate logic.
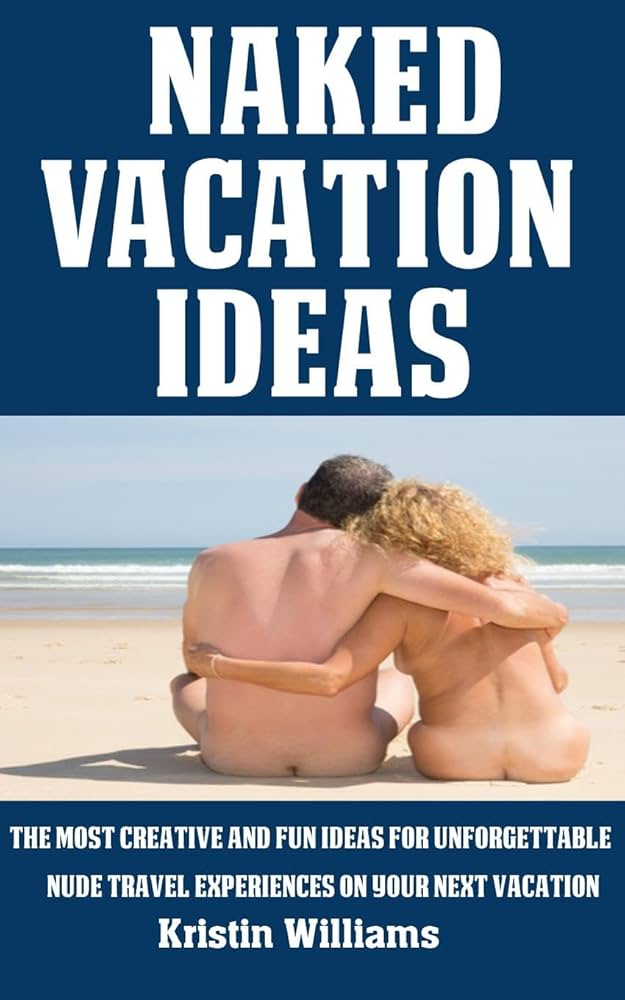
What did I learn? This whole process reinforced the importance of clear code structure and testing. Breaking the problem down into smaller, manageable chunks (finding twins, then eliminating candidates) made it way easier to debug. And all those print statements? Lifesavers!
Next up, I’m thinking of tackling “hidden twins” or maybe even “naked triples.” It’s a Sudoku rabbit hole, I tell ya!