Alright, let’s dive into my “decouple season 2” adventure. It was a bit of a bumpy ride, but hey, that’s how we learn, right?
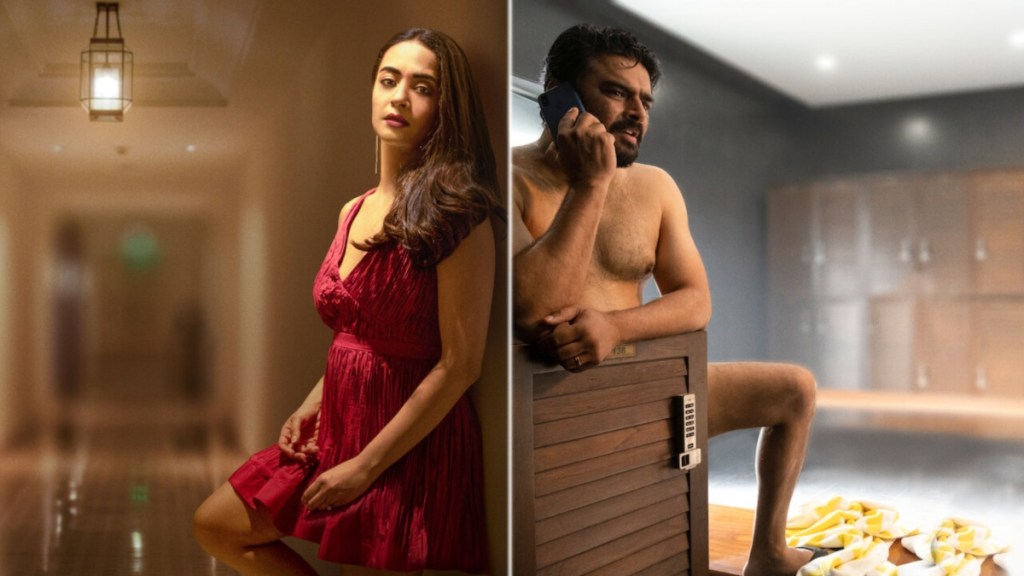
So, the whole thing kicked off because I was wrestling with this monolithic application. You know, the kind where everything’s tangled together like a plate of spaghetti. Making even the smallest change felt like defusing a bomb. I knew I had to do something about it.
The Initial Plan: Microservices, baby! I jumped headfirst into microservices. Thought I’d break down the whole beast into smaller, manageable chunks. I started by identifying the core functionalities that could stand on their own. Think user authentication, payment processing, stuff like that. Each one would be its own little service, with its own database and everything.
The Tech Stack: A bit of this, a bit of that. For the new services, I decided to go with a mix of things. Python with Flask for some, * for others. Figured I’d use Docker to containerize everything and Kubernetes to orchestrate it all. Seemed like a solid plan on paper.
The Actual Process: Oh, the headaches! This is where things got interesting. First off, extracting the functionalities from the monolith was a pain. It was like untangling that plate of spaghetti I mentioned earlier. There were dependencies all over the place, and I had to spend ages refactoring code just to get things to a point where they could be separated.
- Database Troubles: Each microservice needed its own database. That meant migrating data, dealing with schema changes, and ensuring consistency across all the databases. What a mess!
- Communication Chaos: Getting the microservices to talk to each other was another challenge. I tried using REST APIs at first, but that quickly became a bottleneck. Switched to message queues (RabbitMQ) for asynchronous communication. That helped, but added another layer of complexity.
- Deployment Nightmares: Deploying and managing all these microservices on Kubernetes was a whole different ballgame. I had to learn about pods, deployments, services, ingress controllers… the list goes on. There were a few sleepless nights spent debugging YAML files, that’s for sure.
The (Almost) Breaking Point: At one point, I was ready to throw in the towel. The whole thing felt like a bigger mess than the original monolith. Debugging was a nightmare, deployments were slow and painful, and I was spending more time managing infrastructure than writing actual code.
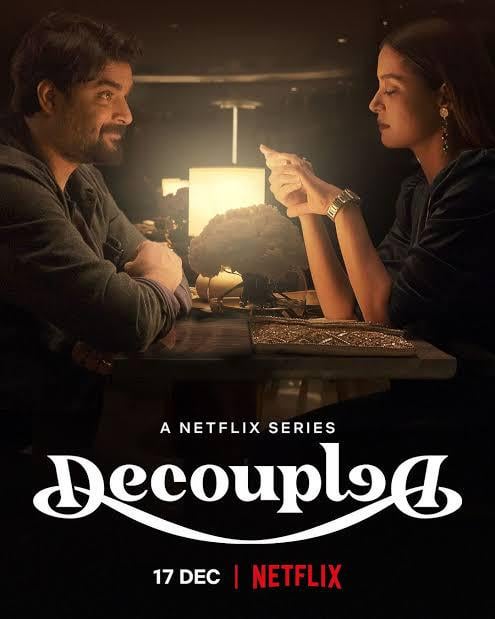
The Turning Point: Back to Basics. I took a step back and realized I was overcomplicating things. I didn’t need to go full-blown microservices right away. I decided to focus on decoupling the application at a higher level, without necessarily creating separate services. I started by introducing clear boundaries between different modules within the monolith.
The New Approach: Modular Monolith. I adopted a modular monolith architecture. The application was still a single deployable unit, but it was organized into distinct modules with well-defined interfaces. This made it easier to understand, test, and modify the code.
How I Did It:
- Defined Clear Boundaries: I used techniques like domain-driven design to identify the different domains within the application and create clear boundaries between them.
- Used Interfaces: I defined interfaces for each module and used dependency injection to wire them together. This allowed me to swap out implementations without affecting other modules.
- Improved Testing: With the code organized into modules, it became much easier to write unit tests and integration tests. This gave me more confidence when making changes.
The Result: A Much Happier Developer. The modular monolith approach worked wonders. The application was still a single deployable unit, but it was much easier to manage and maintain. I could make changes without fear of breaking everything, and the codebase was much more understandable.
Lessons Learned:
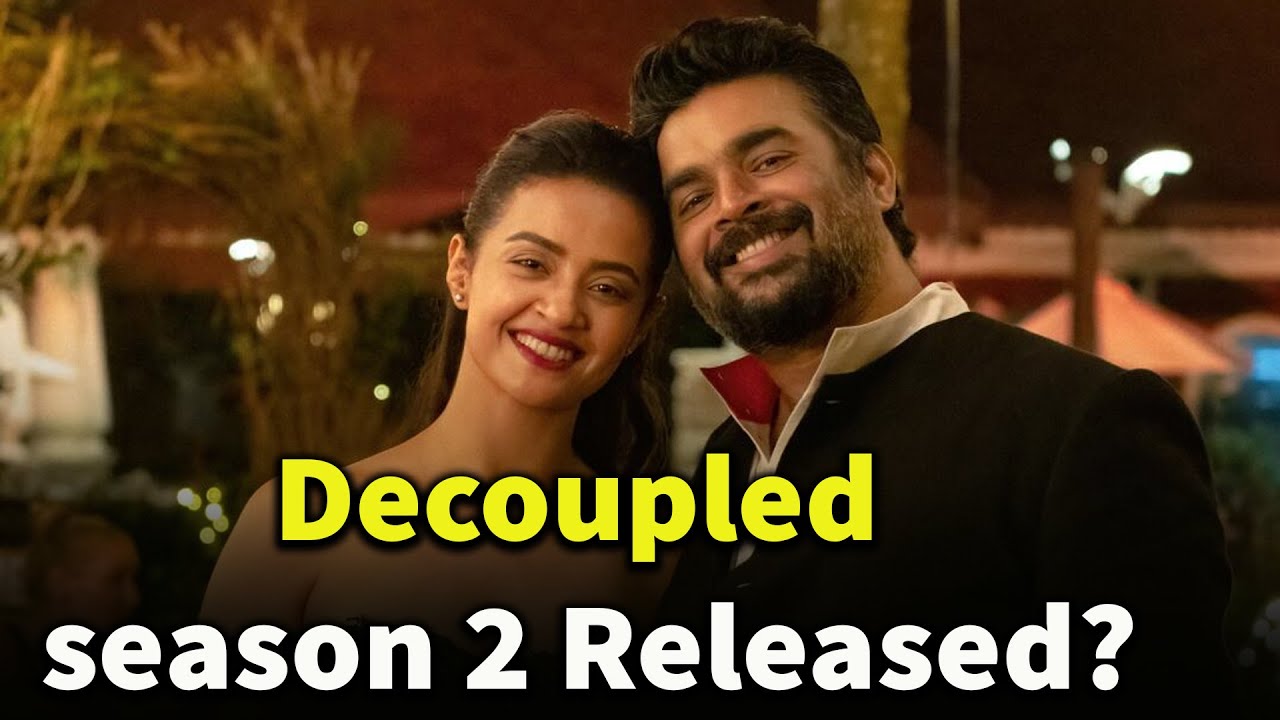
- Don’t over-engineer things: Start simple and gradually add complexity as needed.
- Microservices are not a silver bullet: They can be a great solution for certain problems, but they also add a lot of complexity.
- Modular monoliths can be a good middle ground: They provide many of the benefits of microservices without the added complexity.
So, that’s my “decouple season 2” story. It was a bit of a rollercoaster, but I learned a lot along the way. And now, I have a much better understanding of how to decouple applications without going completely crazy.
Next Steps: I’m planning to gradually migrate some of the modules into separate microservices as needed. But for now, I’m happy with the modular monolith approach. It’s a good balance between simplicity and flexibility.
Hope this helps anyone else struggling with a monolithic application! Good luck!